Unity進階---AssetBundle
阿新 • • 發佈:2018-12-18
AssetBundle的定義和作用: 1,AssetBundle是一個壓縮包包含模型、貼圖、預製體、聲音、甚至整個場景,可以在遊戲執行的時候被載入; 2,AssetBundle自身儲存著互相的依賴關係; 3,壓縮包可以使用LZMA和LZ4壓縮演算法,減少包大小,更快的進行網路傳輸; 4,把一些可以下載內容放在AssetBundle裡面,可以減少安裝包的大小;
AssetBundle使用流程:
- 進行AB包的時候進行屬性設定
- 將設定好的屬性(預製體…) 壓縮成為一個特定的檔案 程式碼壓縮的!!!
- 放置到web/gameserver上面
- 客戶端經過檢驗之後下載
- 下載之後解壓例項化
編譯器擴充套件:
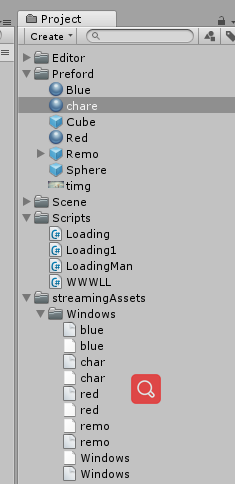
編譯器擴充套件
using UnityEngine;
using UnityEditor;
using System.IO;
public class BuildAssetBundleTools{
[MenuItem("AssetBundleTools/BuildAB")]
public static void Building()
{
string _path = Application.streamingAssetsPath + "\\Windows";
BuildPipeline.BuildAssetBundles(_path, BuildAssetBundleOptions.None, BuildTarget.StandaloneWindows64);
}
}
依賴
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class LoadingMan : MonoBehaviour {
public string Name; //定義一個需要載入或例項化物體的名字
string _path; //路徑
string _manth;
private List<AssetBundle> ManListAB; //定義一個列表 用來儲存載入完畢後的依賴關係
void Start () {
ManListAB = new List<AssetBundle>();
_path = Application.streamingAssetsPath + "/Windows/"; // "/Windows/Name" 寫活了
_manth = Application.streamingAssetsPath + "/Windows/Windows"; //路徑檔案地址
AssetBundle manAB = AssetBundle.LoadFromFile(_manth); //載入路徑
AssetBundleManifest manifest = manAB.LoadAsset<AssetBundleManifest>("AssetBundleManifest"); //Windows所有的依賴關係
string[] depen = manifest.GetAllDependencies(Name); //得到你想要的那個物體的 依賴關係(材質AssetBundle的名稱)
if (depen.Length != 0)
{
foreach (string v in depen) //遍歷string[]裡面的依賴關係
{
//呼叫LoadByName方法 挨個載入依賴關係 並存儲在列表裡
Debug.Log(v);
ManListAB.Add(LoadByName(v));
}
}
//載入物體資訊 並開始例項化
AssetBundle ab = AssetBundle.LoadFromFile(_path + Name); //檔案拼接 拼成完整的路徑地址
GameObject obj = ab.LoadAsset<GameObject>("Remo");
Instantiate(obj);
foreach (var v in ManListAB)
{
v.Unload(false);
}
ab.Unload(false);//必須要有這句話 否則重複載入 報錯
//下面例項化的物體將沒有材質 因為依賴關係被釋放
AssetBundle ab01 = AssetBundle.LoadFromFile(_path + Name);
GameObject obj01 = ab01.LoadAsset<GameObject>("Remo");
Instantiate(obj01, Vector3.one, Quaternion.identity);
}
void Update () {
}
private AssetBundle LoadByName(string name)
{
return AssetBundle.LoadFromFile(_path + name);
}
}
簡單載入
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Loading : MonoBehaviour {
private Vector3 a = new Vector3(3, 0, 0);
// Use this for initialization
void Start () {
string _path = Application.streamingAssetsPath + "\\Windows\\asd";
AssetBundle ab = AssetBundle.LoadFromFile(_path);
if (ab == null)
{
Debug.LogError("載入失敗");
return;
}
GameObject obj = ab.LoadAsset<GameObject>("Cube");
if (obj != null)
{
Instantiate(obj);
}else
{
Debug.LogError("該資源不存在");
}
StartCoroutine("Creat",ab);
}
IEnumerator Creat(AssetBundle ab)
{
yield return new WaitForSeconds(3);
//ab.Unload(false);
yield return new WaitForSeconds(5);
GameObject obj = Instantiate(ab.LoadAsset<GameObject>("Sphere"),a,Quaternion.identity);
}
}
利用WWW載入
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class WWWLL : MonoBehaviour {
// Use this for initialization
void Start () {
string _path = "file://" + Application.streamingAssetsPath + "/Windows/char";
StartCoroutine("Load", _path);
}
IEnumerator Load(string path)
{
using (WWW load01 = new WWW(path))
{
yield return load01;
if (load01 != null)
{
AssetBundle ab = load01.assetBundle;
if (ab != null)
{
GameObject obj = ab.LoadAsset<GameObject>("Capsule");
Instantiate(obj);
}
}else
{
Debug.LogError("載入失敗");
}
}
}