Speed up the Web Development Productivity with Selenium
Speed up the Web Development Productivity with Selenium

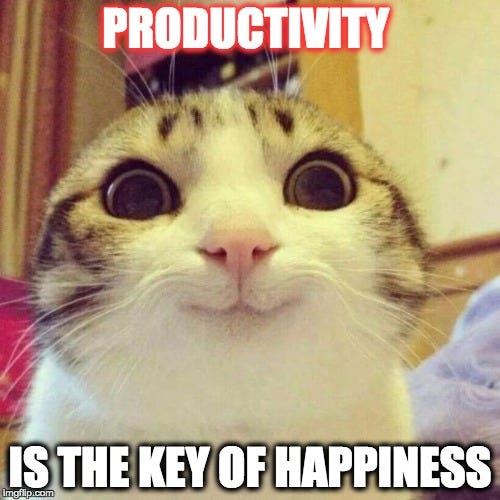
Imagine that you are developing a website, and every time you restart, fixing bugs or adding features. You have go through a repetitive process such as log in with different users, then answer the security question, then click to a few tabs in order to go the place where you want to work.
Each steps may take only a few seconds but for the long run, it will cost you more than that. And I really hate repetition. It’s boring!
And then, there is a tool named Selenium that comes from thin air and save the date — it doesn’t come up from thin air. It’s a suite of tools specially for automating web browsers
In this post, I will use Selenium-Python to boost my web development process. Please read their instruction to install Python and Selenium to your machine before you move forward.
OS: MacOS SierraEditor: Visual Studio CodeProject: AngularJSBrowser: Chrome (remember to install chromedriver)
In short, I will write a small chunk of code in Python, and it will help me to open Chrome browser, log me in with username and password, then answer the security password for me so I won’t have to do it ever again. That’s the basic.
Create your python test file
// test.py
import selenium
from selenium import webdriver
# Using Chrome to open the webbrowser = webdriver.Chrome()
# Open the websitebrowser.get('http://your-website.com')
Test your python file by running:
// python3 if you are using version 3python test.py
Make sure that there is no error and it will open http://your-website.com in a new Chrome browser.
Try to login through your test file
One thing that I notice is that there are lots of websites that doesn’t load automatically, it has to wait for a few seconds before your element appear on the browser. So in your test file, it’s better to wait for the element to show up before testing.
// test.pyimport selenium
from selenium import webdriverfrom selenium.webdriver.support.ui import WebDriverWaitfrom selenium.webdriver.support import expected_conditions as ECfrom selenium.webdriver.common.by import Byfrom selenium.common.exceptions import TimeoutException
# Using Chrome to open the webbrowser = webdriver.Chrome()
# Open the websitebrowser.get('http://your-website.com')
delay = 3 # secondstry: WebDriverWait(browser, delay).until(EC.presence_of_element_located((By.ID, 'username'))) print ("Username Input is ready!")except TimeoutException: print ("Loading took too much time!")
For example, the code above will load the page and wait until the element with Id = username presents. I assume that you know how to get the id for each element, either through your code or through browser inspector.
Now we can automate our login process:
// test.py"""Start to login"""
# select the log Id boxid_box = browser.find_element_by_id('username')
# Send id informationid_box.send_keys('dalenguyen')
# Password enterpass_box = browser.find_element_by_id('password')pass_box.send_keys('123456')
# Find login buttonlogin_button = browser.find_element_by_id('login-submit')login_button.click()
Now, if you run python test.py, it will open a new browser and automatically enters username + password, then log you in.
I think it is enough for you to play around with selenium-python. The application is not limit to just login your account, but you can upload file, drag and drop, go to different tabs or actions as long as you can find the element through their ID, Classname, Tag name, or XPath… You should read their document for your information.
Hope this will help to improve your testing or development process ;)