【LeetCode每天一題】Longest Substring Without Repeating Characters(最長無重復的字串)
阿新 • • 發佈:2019-03-27
bst rate 空間 破解 exp 最大 復雜度 must 判斷字符串
對於這道題最簡單的方法我們可以使用暴力破解法,嘗試所有可能的搜索字串,然後得出最大的不重復字串,但是這種解法復雜度太高,遇到比較長的字符串時,可能直接TimeOut了,所以嘗試有沒有其他解法。 另一種解法就是我們通過設置一個標誌量來表示當前從哪一個位置的字串開始計算的和一個字典輔助空間來記錄字符出現的位置,然後從頭到尾遍歷字串,先判斷字符串是否存在字典中並且當前的下標需要大於等於標誌量,滿足的話取出最大的具體,然後設置當前具體,並且將標誌量移動到字典中第一個重復出現的字符串的下一位。然後繼續執行。
復雜度
時間復雜度為O(n), 空間復雜度為O(n)(字典存儲所耗費的空間較大,也估計為O()) 圖示步驟:
代碼:
Given a string, find the length of the longest substring without repeating characters.
Example 1: Input: "abcabcbb" Output: 3 Explanation: The answer is "abc"
, with the length of 3.
Example 2: Input: "bbbbb" Output: 1 Explanation: The answer is "b"
, with the length of
Example 3: Input: "pwwkew" Output: 3 Explanation: The answer is "wke"
, with the length of 3.
Note that the answer must be a substring, "pwke"
is a subsequence and not a substring.
對於這道題最簡單的方法我們可以使用暴力破解法,嘗試所有可能的搜索字串,然後得出最大的不重復字串,但是這種解法復雜度太高,遇到比較長的字符串時,可能直接TimeOut了,所以嘗試有沒有其他解法。 另一種解法就是我們通過設置一個標誌量來表示當前從哪一個位置的字串開始計算的和一個字典輔助空間來記錄字符出現的位置,然後從頭到尾遍歷字串,先判斷字符串是否存在字典中並且當前的下標需要大於等於標誌量,滿足的話取出最大的具體,然後設置當前具體,並且將標誌量移動到字典中第一個重復出現的字符串的下一位。然後繼續執行。
時間復雜度為O(n), 空間復雜度為O(n)(字典存儲所耗費的空間較大,也估計為O()) 圖示步驟:
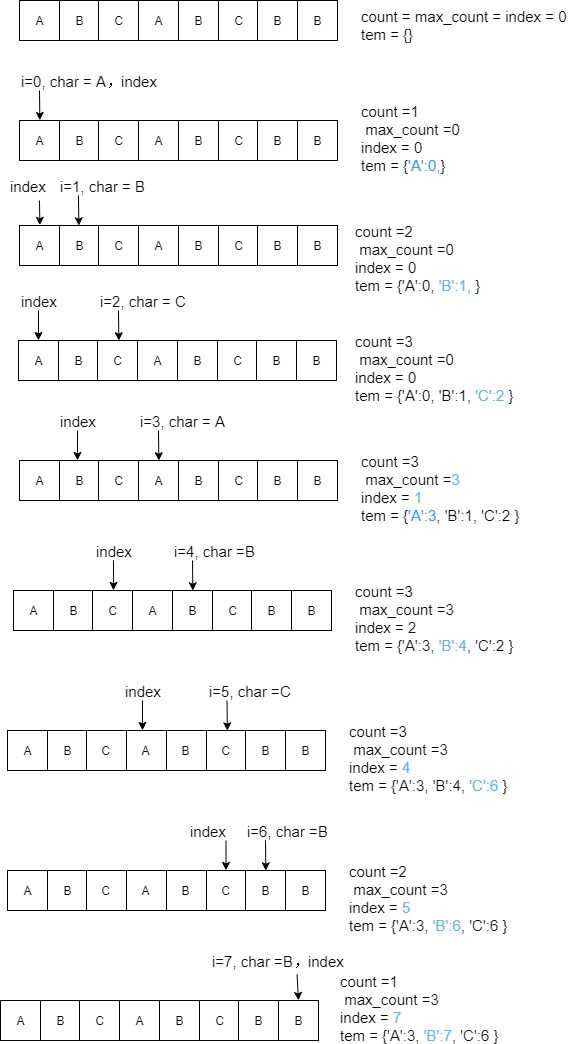
1 class Solution(object):
2 def lengthOfLongestSubstring(self, s):
3 """
4 :type s: str
5 :rtype: int
6 """
7 if len(s) < 2:
8 return 1 if len(s) == 1 else 0
9 index, max_count = 0, 0 # 設置標誌量和最大長不重復字段的數目
10 tem_dict, count = {}, 0 # 設置輔助空間字典和當前的技術器
11 for i, char in enumerate(s): # 沖頭開始遍歷
12 if char in tem_dict and tem_dict[char] >= index: # 如果當前字符在字典中並且字典中的下標大於等於標誌量下標(標誌量表示從哪一個字符開始計算的)
13 max_count =max(count,max_count) # 求出最大的數
14 count = i - tem_dict[char] # 算出第一個出現重復的字符串當第二次出現時的距離數。
15 index = tem_dict[char]+1 # 將標誌量設置到第一次出現重復字符串的下一個。
16 else:
17 count += 1 # 無重復字符出現,計數加1
18 tem_dict[char] = i # 記錄當前字符串下標
19 return max(count, max_count) # 返回最大的距離數
【LeetCode每天一題】Longest Substring Without Repeating Characters(最長無重復的字串)