【LeetCode每天一題】Remove Element(移除指定的元素)
Given an array nums and a value val, remove all instances of that value in-place and return the new length.Do not allocate extra space for another array, you must do this by modifying the input array in-place with O(1) extra memory. The order of elements can be changed. It doesn‘t matter what you leave beyond the new length.
Example 1: Given nums = [3,2,2,3], val = 3
Your function should return length = 2, with the first two elements of nums being 2.It doesn‘t matter what you leave beyond the returned length.
Example 2: Given nums = [0,1,2,2,3,0,4,2], val = 2,
Your function should return length = 5
nums
containing 0
, 1
, 3
, 0
, and 4.Note that the order of those five elements can be arbitrary. It doesn‘t matter what values are set beyond the returned length.
思路
因為我經常使用python解題,當我看到這道題之後使用python中列表自帶的pop()方法來解決的,就是從頭遍歷到尾,如果相等的元素我們就直接彈出。遍歷到尾部結束。時間復雜度為O(n),空間復雜度為O(1)。
如果我們不使用pop()方法,有沒有其他方法可以解決?我想到了兩個指針的方法分別指向頭和尾,如果遇到和val相等的元素將尾指針指向的元素賦值到頭指針的位置。直到頭和尾指針相遇結束。時間復雜度為O(n), 空間復雜度為O(1).
第二種思路圖示
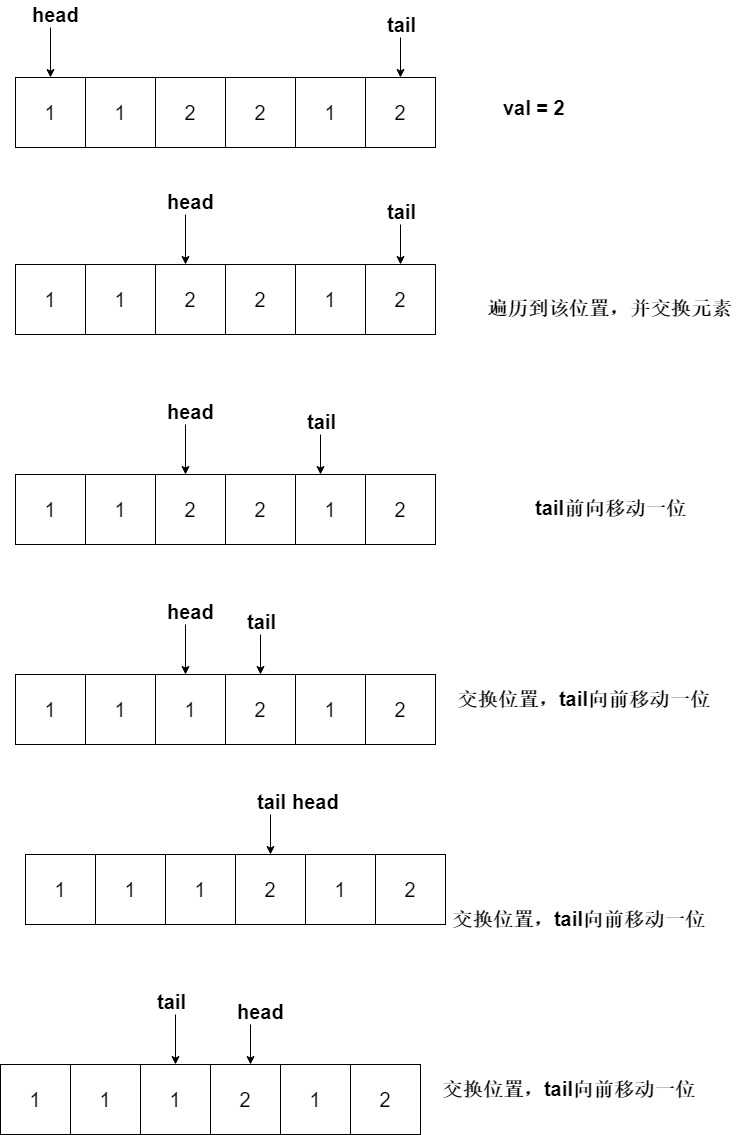
第一種思路解決代碼
1 class Solution(object):
2 def removeElement(self, nums, val):
3 """
4 :type nums: List[int]
5 :type val: int
6 :rtype: int
7 """
8 if len(nums) < 1: # 沒有元素直接彈出
9 return 0
10 i,leng = 0, len(nums)
11
12 while i < len(nums):
13 if nums[i] == val: # 相等就彈出,
14 nums.pop(i)
15 leng -= 1
16 else:
17 i +=1
18 return len(nums)
第二種思路解決代碼
1 class Solution(object):
2 def removeElement(self, nums, val):
3 """
4 :type nums: List[int]
5 :type val: int
6 :rtype: int
7 """
8 if len(nums) < 1:
9 return 0
10 i,leng = 0, len(nums)-1
11 while i <= leng:
12 if nums[i] == val:
13 nums[i] = nums[leng] # 將尾部的元素進行賦值。
14 leng -= 1
15 else:
16 i+= 1
17 return leng+1
【LeetCode每天一題】Remove Element(移除指定的元素)