【HDFS API編程】第一個應用程序的開發-創建文件夾
/**
* 使用Java API操作HDFS文件系統
* 關鍵點:
* 1)創建 Configuration
* 2)獲取 FileSystem
* 3)...剩下的就是 HDFS API的操作了
*/
先上代碼
1 public class HDFSApp { 2 public static void main(String[] args) throws Exception { 3 Configuration configuration = new Configuration(); 4 5 FileSystem fileSystem = FileSystem.get(newURI("hdfs://hadoop000:8020"),configuration,"hadoop"); 6 7 Path path = new Path("/hdfsapi/test"); 8 boolean result = fileSystem.mkdirs(path); 9 System.out.println(result); 10 } 11 }
對於方法的源碼是我們在學習的時候必須的,我們可以按住Ctrl然後點擊對應的方法類名進去進行源碼的下載。
首先要對HDFS進行操作我們就必須獲取FileSystem,
Ctrl點進FileSystem源碼,我們能看到最頂部的註釋介紹(附上了中文翻譯):
1 /**************************************************************** 2 * An abstract base class for a fairly generic filesystem. It 3 * may be implemented as a distributed filesystem, or as a "local" 4 * one that reflects the locally-connected disk. The local version 5 * exists for small Hadoop instances and for testing.6 * 7 * <p> 8 * 9 * All user code that may potentially use the Hadoop Distributed 10 * File System should be written to use a FileSystem object. The 11 * Hadoop DFS is a multi-machine system that appears as a single 12 * disk. It‘s useful because of its fault tolerance and potentially 13 * very large capacity. 14 * 15 * <p> 16 * The local implementation is {@link LocalFileSystem} and distributed 17 * implementation is DistributedFileSystem. 18 *****************************************************************/ 19 /********************************************** 20 21 *一個相當通用的文件系統的抽象基類。它可以實現為分布式文件系統,也可以實現為“本地” 22 *反映本地連接磁盤的磁盤。本地版本 23 *存在於小型Hadoop實例和測試中。 24 *所有可能使用Hadoop分布式的用戶代碼 25 *應該寫入文件系統以使用文件系統對象。這個Hadoop DFS是一個多機系統,顯示為單個磁盤。它是有用的,因為它的容錯性和潛在的容量很大。 26 *< P> 27 *本地實現是@link localfilesystem和分布式的 28 *實現是分布式文件系統。 29 ***********************************************/
寫作的開門見山,第一句話能夠概括全文中心---> 這是 一個相當通用的文件系統的抽象基類。,所以我們使用Java API操作HDFS文件系統需要獲取FileSystem。
使用FileSystem的get方法獲取fileSystem,FileSystem有三種get方法:
不知道怎麽用?哪裏不會Ctrl點哪裏!
首先看只有一個參數的get方法:get(Configuration conf):
1 /** 2 * Returns the configured filesystem implementation. 3 * @param conf the configuration to use 4 */ 5 /** 6 *返回配置的文件系統實現。 7 *@參數conf 要使用的配置 8 */ 9 public static FileSystem get(Configuration conf) throws IOException { 10 return get(getDefaultUri(conf), conf); 11 }
從源碼的介紹中可以知道 Configuration 是某種配置,具體是什麽?Ctrl點一點:
Provides access to configuration parameters.//提供對配置參數的訪問。
源碼中configuration的註釋介紹第一句已經介紹了這是對配置參數的訪問,所以,使用Java API操作HDFS文件系統需要創建configuration:Configuration configuration = new Configuration();
跳過兩個參數的get方法,我們來看包含三個參數的get方法,Ctrl 點進去:
1 /** 2 * Get a filesystem instance based on the uri, the passed 3 * configuration and the user 4 * @param uri of the filesystem 5 * @param conf the configuration to use 6 * @param user to perform the get as 7 * @return the filesystem instance 8 * @throws IOException 9 * @throws InterruptedException 10 */ 11 /** 12 *獲取基於URI的文件系統實例,配置和用戶 13 *@參數uri 文件系統的uri 14 *@參數conf 要使用的配置 15 *@參數user 要執行get as的用戶 16 *@返回文件系統實例 17 *@引發IOException 18 *@引發InterruptedException 19 */ 20 public static FileSystem get(final URI uri, final Configuration conf, 21 final String user) throws IOException, InterruptedException { 22 String ticketCachePath = 23 conf.get(CommonConfigurationKeys.KERBEROS_TICKET_CACHE_PATH); 24 UserGroupInformation ugi = 25 UserGroupInformation.getBestUGI(ticketCachePath, user); 26 return ugi.doAs(new PrivilegedExceptionAction<FileSystem>() { 27 @Override 28 public FileSystem run() throws IOException { 29 return get(uri, conf); 30 } 31 }); 32 }
重點看方法上面的註釋,介紹了方法的功能、參數解釋、返回值以及可能引發的異常。
我們要對HDFS操作,是不是需要知道我們要對哪個HDFS操作(URI),是不是要指定用什麽樣的配置參數進行操作(configuration),是不是需要確認是用什麽用戶進行操作(user),這樣我們才能夠準確地使用正確的用戶以及正確的配置訪問正確的HDFS。
此時我們拿到的fileSystem已經是我們需要的fileSystem了,那麽剩下的操作就是對HDFS API的操作了,例如我們創建一個文件夾:
使用fileSystem的mkdirs方法,mkdirs方法不知道怎麽用?Ctrl點!
1 /** 2 * Call {@link #mkdirs(Path, FsPermission)} with default permission. 3 */ 4 public boolean mkdirs(Path f) throws IOException { 5 return mkdirs(f, FsPermission.getDirDefault()); 6 }
我們可以看到mkdir方法接收的參數是一個Path路徑,返回值是boolean類型判斷是否創建成功,所以我們可以寫:
Path path = new Path("/hdfsapi/test");//創建一個Path
boolean result = fileSystem.mkdirs(path);
System.out.println(result);//輸出result查看是否創建成功
此時我們可以通過終端控制臺進行查看文件夾是否創建成功:


當然也可以通過瀏覽器查看是否創建成功:輸入 地址IP:50070 回車, 例如 192.168.42.110:50070 回車,
進去點擊Utilities下拉列表的Browse the fiel system,點Go!就能看到:
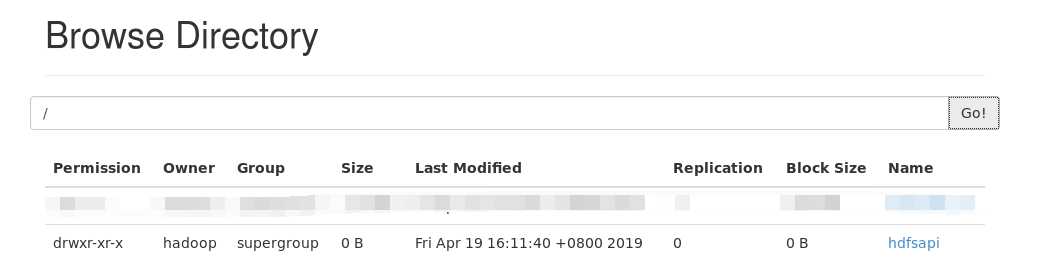
點進去hdfsapi:
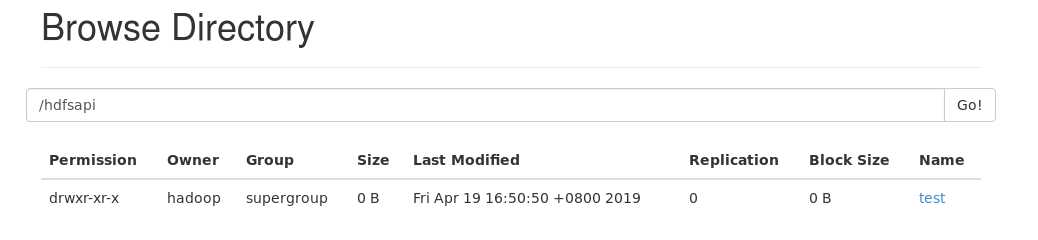
操作成功。
【HDFS API編程】第一個應用程序的開發-創建文件夾