JS實現滾動區域觸底事件
阿新 • • 發佈:2020-03-21
# 效果
貼上效果展示:
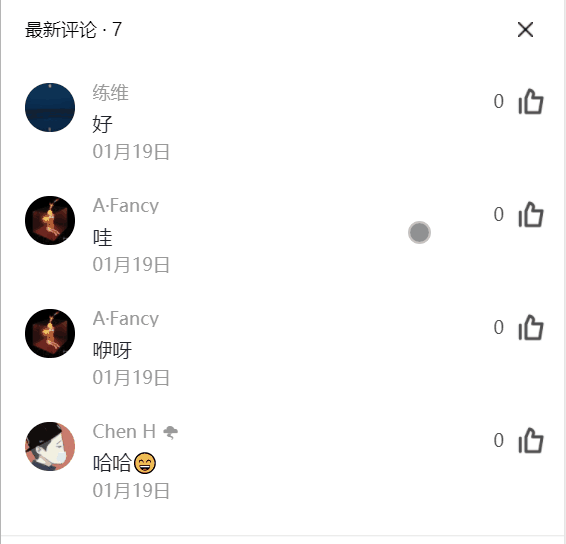
# 實現思路
樣式方面不多贅述,滾動區域是給固定高度,設定 `overflow-y: auto` 來實現。
接下來看看js方面的實現,其實也很簡單,觸發的條件是: `可視高度` + `滾動距離` >= `實際高度` 。例子我會使用`vue`來實現,和原生實現是一樣的。
* 可視高度(offsetHeight):通過 `dom` 的 `offsetHeight` 獲得,表示區域固定的高度。這裡我推薦通過 `getBoundingClientRect()` 來獲取高度,因為使用前者會引起瀏覽器迴流,造成一些效能問題。
* 滾動高度(scrollTop):滾動事件中通過 `e.target.scrollTop` 獲取,表示滾動條距離頂部的px
* 實際高度(scrollHeight):通過 `dom` 的 `scrollHeight` 獲得,表示區域內所有內容的高度(包括滾動距離),也就是實際高度
# 基礎實現
``` js
onScroll(e) {
let scrollTop = e.target.scrollTop
let scrollHeight = e.target.scrollHeight
let offsetHeight = Math.ceil(e.target.getBoundingClientRect().height)
let currentHeight = scrollTop + offsetHeight
if (currentHeight >= scrollHeight) {
console.log('觸底')
}
}
```
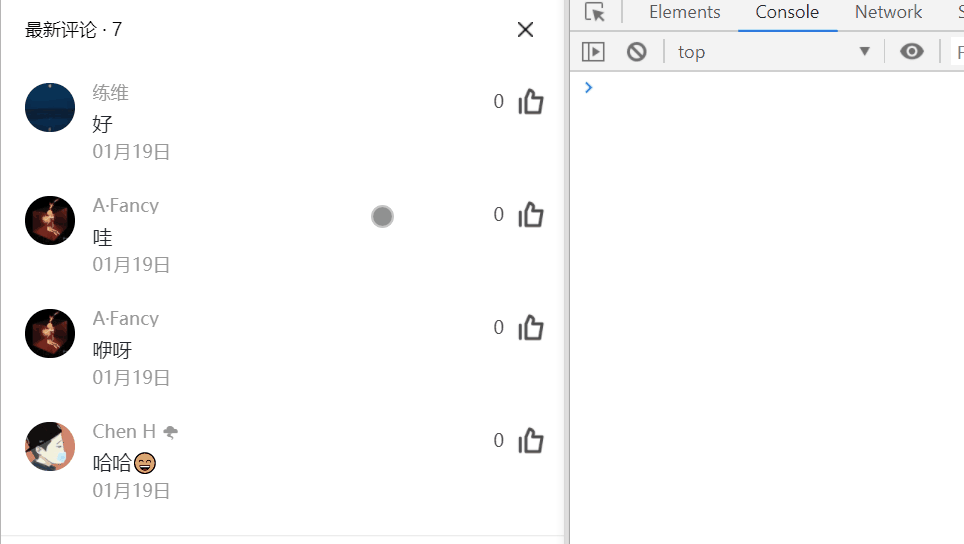
so easy~
# 加點細節
加點細節,現在我們希望是離底部一定距離就觸發事件,而不是等到完全觸底。如果你做過小程式,這和`onReachBottom`差不多的意思。
宣告一個離底部的距離變數`reachBottomDistance`
這時候觸發條件:`可視高度` + `滾動距離` + `reachBottomDistance` >= `實際高度`
```js
export default {
data(){
return {
reachBottomDistance: 100
}
},
methods: {
onScroll(e) {
let scrollTop = e.target.scrollTop
let scrollHeight = e.target.scrollHeight
let offsetHeight = Math.ceil(e.target.getBoundingClientRect().height)
let currentHeight = scrollTop + offsetHeight + this.reachBottomDistance
if (currentHeight >= scrollHeight) {
console.log('觸底')
}
}
}
}
```
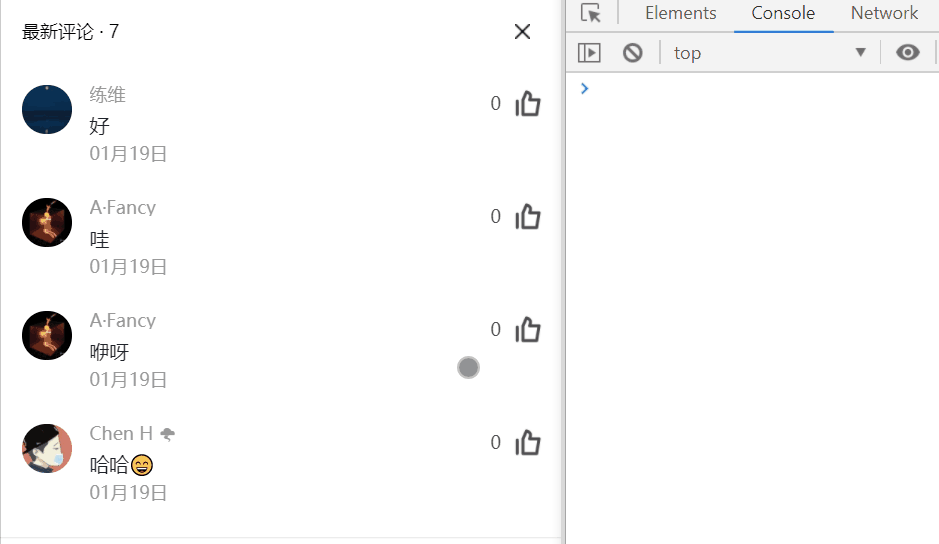
在距離底部100px時成功觸發事件,但由於100px往下的區域是符合條件的,會導致一直觸發,這不是我們想要的。
接下來做一些處理,讓其進入後只觸發一次:
```js
export default {
data(){
return {
isReachBottom: false,
reachBottomDistance: 100
}
},
methods: {
onScroll(e) {
let scrollTop = e.target.scrollTop
let scrollHeight = e.target.scrollHeight
let offsetHeight = Math.ceil(e.target.getBoundingClientRect().height)
let currentHeight = scrollTop + offsetHeight + this.reachBottomDistance
if(currentHeight < scrollHeight && this.isReachBottom){
this.isReachBottom = false
}
if(this.isReachBottom){
return
}
if (currentHeight >= scrollHeight) {
this.isReachBottom = true
console.log('觸底')
}
}
}
}
```
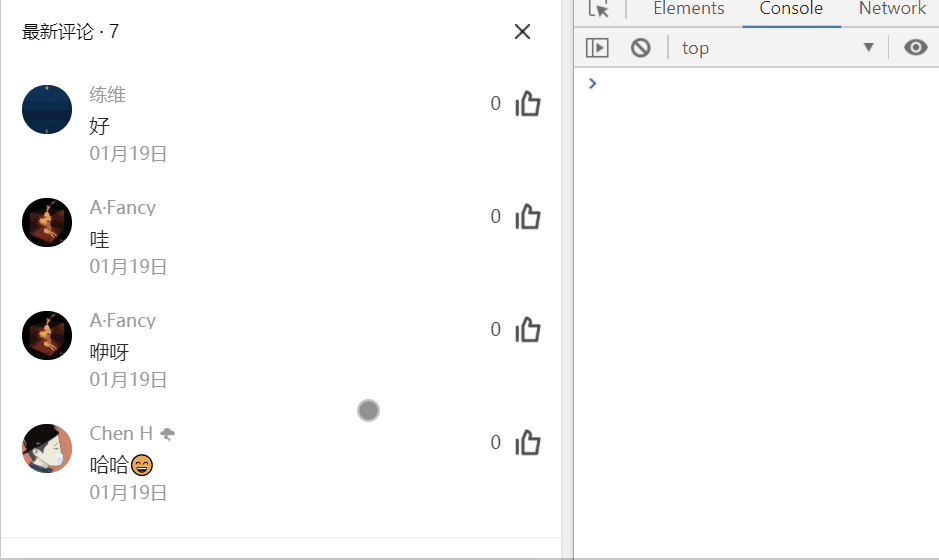
# 優化
實時去獲取位置資訊稍微會損耗效能,我們應該把不變的快取起來,只實時獲取可變的部分
```js
export default {
data(){
return {
isReachBottom: false,
reachBottomDistance: 100
scrollHeight: 0,
offsetHeight: 0,
}
},
mounted(){
// 頁面載入完成後 將高度儲存起來
let dom = document.querySelector('.comment-area .comment-list')
this.scrollHeight = dom.scrollHeight
this.offsetHeight = Math.ceil(dom.getBoundingClientRect().height)
},
methods: {
onScroll(e) {
let scrollTop = e.target.scrollTop
let currentHeight = scrollTop + this.offsetHeight + this.reachBottomDistance
if(currentHeight < this.scrollHeight && this.isReachBottom){
this.isReachBottom = false
}
if(this.isReachBottom){
return
}
if (currentHeight >= this.scrollHeight) {
this.isReachBottom = true
console.log('觸底')
}
}
}
}
```
實現到這裡就告一段落,如果你有更好的思路和值得改進的地方,歡