一句話+兩張圖搞定JDK1.7HashMap,剩下湊字數
阿新 • • 發佈:2020-05-16
# JDK1.7 HashMap一探究竟
HashMap很簡單,原理一看散列表,實際陣列+連結串列;Hash找索引.索引若為null,while下一個.Hash對對碰,連結串列依次查.載入因子.75,剩下無腦擴陣列.
### 開局兩張圖,剩下全靠編
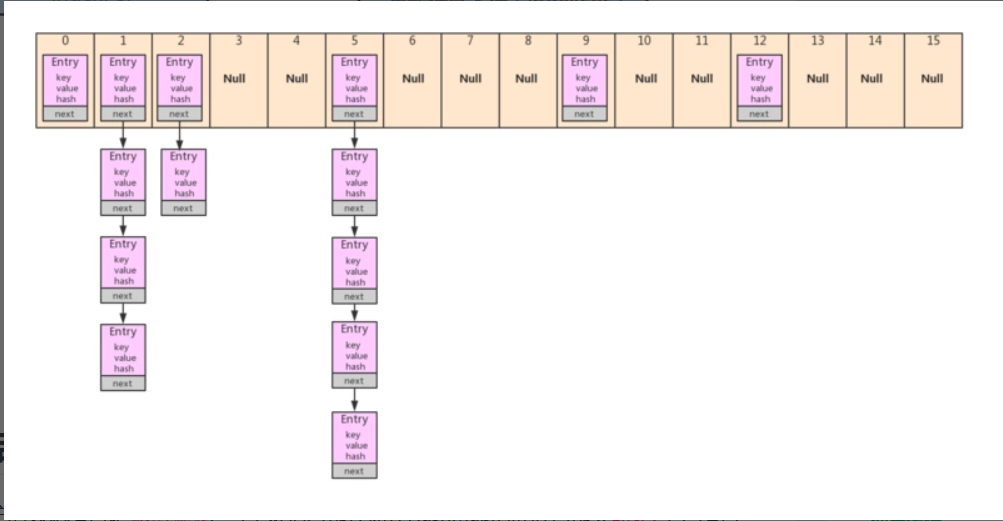
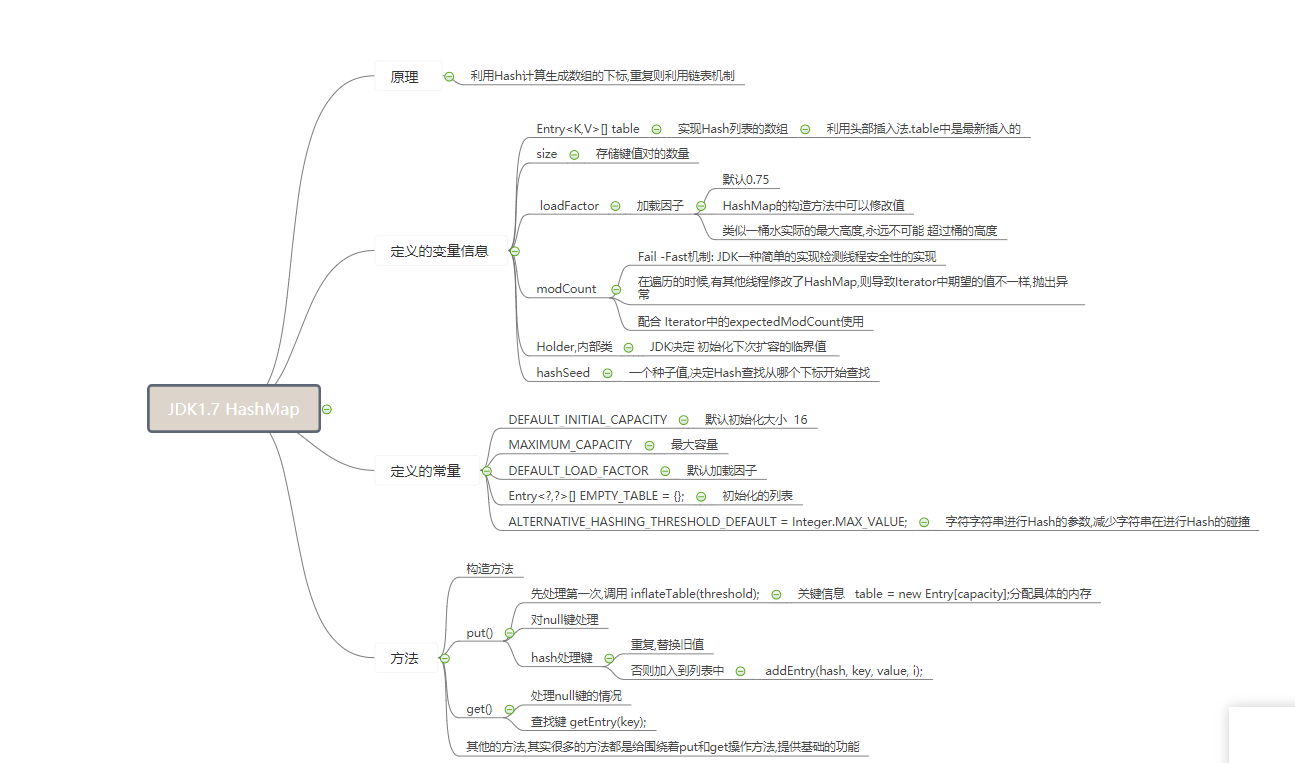
### JDK1.7的HashMap
```java
public class HashMap
extends AbstractMap
implements Map, Cloneable, Serializable
```
+ AbstractMap 對一些簡單的功能的實現
+ Map介面,定義了一組通用的Map操作的方法
+ Cloneable介面,可以拷貝
+ Serializable 可序列化
### 定義的常量
```java
static final int DEFAULT_INITIAL_CAPACITY = 1 << 4; // aka 16 預設初始化大小 10000B
static final int MAXIMUM_CAPACITY = 1 << 30; //最大的容量 2的20次方
static final float DEFAULT_LOAD_FACTOR = 0.75f; //影響因子 0.75 影響因子是雜湊表在其容量自動增加之前可以達到多滿的一種尺度。當雜湊表中的條目 數超出了載入因子與當前容量的乘積時,通過呼叫 rehash 方法將容量翻倍。
static final Entry,?>[] EMPTY_TABLE = {};// 字面意思雜湊表的入口陣列.
static final int ALTERNATIVE_HASHING_THRESHOLD_DEFAULT = Integer.MAX_VALUE; //表示在對字串鍵(即key為String型別)的HashMap應用備選雜湊函式時HashMap的條目數量的預設閾值。備選雜湊函式的使用可以減少由於對字串鍵進行弱雜湊碼計算時的碰撞概率。
```
### 定義的變數
```java
transient Entry[] table = (Entry[]) EMPTY_TABLE; //不可序列化的雜湊表,初始化為上面空的雜湊表
transient int size; //雜湊表中儲存的資料
int threshold; // 下次擴容的臨界值,size>=threshold就會擴容
final float loadFactor; //影響因子
transient int modCount; //修改的次數
private static class Holder {...} //它的作用是在虛擬機器啟動後根據jdk.map.althashing.threshold和ALTERNATIVE_HASHING_THRESHOLD_DEFAULT初始化ALTERNATIVE_HASHING_THRESHOLD
transient int hashSeed = 0; //seed 種子值
```
### HashMap的構造方法
1.
```java
public HashMap(int initialCapacity, float loadFactor){
this.loadFactor = loadFactor;
threshold = initialCapacity; //threshold 下次擴容的臨界值也設定為 initialCapacity
init(); //一個空方法的實現
}
```
2.
```java
public HashMap(int initialCapacity){
this(initialCapacity, DEFAULT_LOAD_FACTOR);
}
```
3.
```java
public HashMap() {
this(DEFAULT_INITIAL_CAPACITY, DEFAULT_LOAD_FACTOR);
}
```
4.
```java
public HashMap(Map extends K, ? extends V> m) { //用Map初始化
this(Math.max((int) (m.size() / DEFAULT_LOAD_FACTOR) + 1,
DEFAULT_INITIAL_CAPACITY), DEFAULT_LOAD_FACTOR);
inflateTable(threshold);
putAllForCreate(m); //通過forEach,將複製到新的HashMap中
}
```
```java
Math.max((int) (m.size() / DEFAULT_LOAD_FACTOR) + 1,
DEFAULT_INITIAL_CAPACITY) //保證了map構成新的HashMap的時候容量,計算Factor後小於對應的預設因子.
```
### 真正存放鍵值的內部類
```java
static class Entry implements Map.Entry {
final K key;
V value;
Entry next; //一個典型的連結串列的實現.單向連結串列
int hash;
// 建構函式
Entry(int h, K k, V v, Entry n) {
value = v;
next = n;
key = k;
hash = h;
}
//... 一些比較普遍方法的實現
//Entry中的自己的Hash的實現
public final int hashCode() {
return Objects.hashCode(getKey()) ^ Objects.hashCode(getValue());
}
}
```
### 雜湊的計算方法
```java
final int hash(Object k) {
int h = hashSeed; //h為Hash的隨機種子值
if (0 != h && k instanceof String) {
return sun.misc.Hashing.stringHash32((String) k);
} //生成對應字串的雜湊值
h ^= k.hashCode(); //hashCode之後異或
// This function ensures that hashCodes that differ only by
// constant multiples at each bit position have a bounded
// number of collisions (approximately 8 at default load factor).
h ^= (h >>> 20) ^ (h >>> 12); //最前面的12位和最後面的12位進行異或. 在與h異或,得到仍是一個32位的值
return h ^ (h >>> 7) ^ (h >>> 4);
}
```
### 計算儲存下標(indexFor)
```java
/**
* Returns index for hash code h.
*/
static int indexFor(int h, int length) {
// assert Integer.bitCount(length) == 1 : "length must be a non-zero power of 2";
return h & (length-1); //對hash進行與運算,得到對應的儲存的下標
}
```
### get方法的實現
```java
public V get(Object key) {
if (key == null) //對應鍵為null的情況
return getForNullKey();
//普通的情況
Entry entry = getEntry(key);
return null == entry ? null : entry.getValue();
}
```
### 對null鍵處理的函式
這是一個私有方法,方便其他函式的呼叫
``` java
private V getForNullKey() {
if (size == 0) {
return null;
}
for (Entry e = table[0]; e != null; e = e.next) {
if (e.key == null)
return e.value;
}
return null;
}
```
### 獲得鍵值對
```java
final Entry getEntry(Object key) {
if (size == 0) {
return null;
}
int hash = (key == null) ? 0 : hash(key);
for (Entry e = table[indexFor(hash, table.length)];
e != null;
e = e.next) {
Object k;
if (e.hash == hash &&
((k = e.key) == key || (key != null && key.equals(k))))
return e;
}
return null;
}
```
### put方法
```java
public V put(K key, V value) {
if (table == EMPTY_TABLE) {
inflateTable(threshold); //inflateTable方法就是建立雜湊表,分配表記憶體空間的操作(inflate翻譯為“膨脹”的意思,後面會詳述)。但是指定初始容量和負載因子的構造方法並沒有馬上呼叫inflateTable。
}
if (key == null)
return putForNullKey(value);
int hash = hash(key);
int i = indexFor(hash, table.length);
for (Entry e = table[i]; e != null; e = e.next) {
Object k;
if (e.hash == hash && ((k = e.key) == key || key.equals(k))) {//出現重複的值會覆蓋
V oldValue = e.value;
e.value = value;
e.recordAccess(this);
return oldValue;
}
}
modCount++;
addEntry(hash, key, value, i);
return null;
```
### put中的子方法addEntry
```java
void addEntry(int hash, K key, V value, int bucketIndex) {
if ((size >= threshold) && (null != table[bucketIndex])) {
resize(2 * table.length);// 容器當前存放的鍵值對數量是否達到了設定的擴容閾值,如果達到了就擴容2倍。擴容後重新計算雜湊碼,並根據新雜湊碼和新陣列長度重新計算儲存位置。做好潛質處理後,就呼叫createEntry新增一個Entry
hash = (null != key) ? hash(key) : 0;
bucketIndex = indexFor(hash, table.length);
}
createEntry(hash, key, value, bucketIndex);
}
```
### transfer 方法 實現連結串列的擴容中的具體複製
```java
void transfer(Entry[] newTable, boolean rehash) {
int newCapacity = newTable.length; //擴容後的處理
for (Entry e : table) {
while(null != e) {
Entry next = e.next;
if (rehash) {
e.hash = null == e.key ? 0 : hash(e.key);
}
int i = indexFor(e.hash, newCapacity);
e.next = newTable[i];
newTable[i] = e;
e = next; //連結串列成為了倒序
}
}
}
```
### addEntry 中的createEntry方法
```java
void createEntry(int hash, K key, V value, int bucketIndex) {
Entry e = table[bucketIndex]; //入口
table[bucketIndex] = new Entry<>(hash, key, value, e); //讓該節點替代入口地址,原來的連結串列連線在後面.就是所謂的頭插法
size++;
}
```
### 對 threshold的處理
連結串列的容量擴充為2的冪次. 調整 threshold 方法
```java
private void inflateTable(int toSize) {
// Find a power of 2 >= toSize
int capacity = roundUpToPowerOf2(toSize); //實現了增長為2的冪運算. 實現也比較簡單
threshold = (int) Math.min(capacity * loadFactor, MAXIMUM_CAPACITY + 1);
table = new Entry[capacity];
initHashSeedAsNeeded(capacity);
}
```
### HashMap的遍歷
上面HashMap的基本操作已經完成了.下面就是一些對於Iterator介面的實現
```java
private abstract class HashIterator implements Iterator {
Entry next; // next entry to return
int expectedModCount; // For fast-fail
int index; // current slot
Entry current; // current entry
}
```
Fail-Fast 機制
我們知道 java.util.HashMap 不是執行緒安全的,因此如果在使用迭代器的過程中有其他執行緒修改了map,那麼將丟擲ConcurrentModificationException,這就是所謂fail-fast策略。這一策略在原始碼中的實現是通過 modCount 域,modCount 顧名思義就是修改次數,對HashMap 內容的修改都將增加這個值,那麼在迭代器初始化過程中會將這個值賦給迭代器的 expectedModCount。在迭代過程中,判斷 modCount 跟 expectedModCount 是否相等,如果不相等就表示已經有其他執行緒修改了 Map:注意到 modCount 宣告為 volatile,保證執行緒之間修改的可見性。
### 構造方法
```java
HashIterator() {
expectedModCount = modCount;
if (size > 0) { // advance to first entry
Entry[] t = table;
while (index < t.length && (next = t[index++]) == null)
; //在Hash計算的時候會有部分的表為空,找到一個不為空的值
}
}
```
### hasNext() 和 nextEntry()
```java
public final boolean hasNext() {
return next != null; //第一次判斷的是否不為空
}
final Entry nextEntry() {
if (modCount != expectedModCount)
throw new ConcurrentModificationException();
Entry e = next;
if (e == null)
throw new NoSuchElementException();
if ((next = e.next) == null) {
Entry[] t = table;
while (index < t.length && (next = t[index++]) == null)
; //同樣利用迴圈找到寫一個對應的連結串列節點
}
current = e;
return e;
}
```
### 關於多執行緒的問題
假設執行緒A和執行緒B對一個共享的HashMap同時put一個值. put後發現需要擴容,擴容後進行記憶體拷貝`執行transfer方法`.那麼必定出現迴圈連結串列.以後`get()` 的時候出現死迴圈.
### 參考部落格
[理解HashMap](https://segmentfault.com/a/1190000018520768) 老哥寫的比我好lt.com/a/1190000018520768) 老哥寫的比我好