POJ 3498 March of the Penguins(網絡流+枚舉)
題目鏈接:http://poj.org/problem?id=3498
題目:
Description
Somewhere near the south pole, a number of penguins are standing on a number of ice floes. Being social animals, the penguins would like to get together, all on the same floe. The penguins do not want to get wet, so they have use their limited jump distance to get together by jumping from piece to piece. However, temperatures have been high lately, and the floes are showing cracks, and they get damaged further by the force needed to jump to another floe. Fortunately the penguins are real experts on cracking ice floes, and know exactly how many times a penguin can jump off each floe before it disintegrates and disappears. Landing on an ice floe does not damage it. You have to help the penguins find all floes where they can meet.
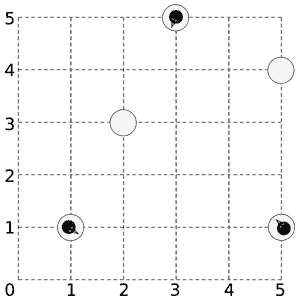
A sample layout of ice floes with 3 penguins on them.
Input
On the first line one positive number: the number of testcases, at most 100. After that per testcase:
-
One line with the integer N (1 ≤ N ≤ 100) and a floating-point number D (0 ≤ D ≤ 100 000), denoting the number of ice pieces and the maximum distance a penguin can jump.
-
N lines, each line containing xi, yi, ni and mi, denoting for each ice piece its X and Y coordinate, the number of penguins on it and the maximum number of times a penguin can jump off this piece before it disappears (−10 000 ≤ xi, yi≤ 10 000, 0 ≤ ni ≤ 10, 1 ≤ mi ≤ 200).
Output
Per testcase:
- One line containing a space-separated list of 0-based indices of the pieces on which all penguins can meet. If no such piece exists, output a line with the single number −1.
Sample Input
2 5 3.5 1 1 1 1 2 3 0 1 3 5 1 1 5 1 1 1 5 4 0 1 3 1.1 -1 0 5 10 0 0 3 9 2 0 1 1
Sample Output
1 2 4 -1
題意:n只企鵝跳冰塊,每只最多跳d米,並且每只企鵝從當前冰塊跳到另一個冰塊上,當前冰塊的壽命-1。問所有企鵝能否跳到同一塊冰塊上,如果可能的話,列舉出所有可能性。
題解:註意題目中冰塊下標是從0開始的。構圖:源點S(0點),1-n枚舉每個終點,把每個冰塊所在的點分割成i(入口)和i+n(出口)兩個點。S與i點連接,容量為該冰塊上
原有企鵝的數目;i與i+n連接,容量為冰塊的壽命;如果i冰塊能夠跳到j冰塊,i+n和j連接,容量為INF。圖構造好直接跑Dinic就可以啦。
1 //POJ 3498 2 #include <queue> 3 #include <cstdio> 4 #include <cstring> 5 #include <iostream> 6 #include <algorithm> 7 using namespace std; 8 9 const int N=233; 10 const int M=2*N*N; 11 const int INF=0x3f3f3f3f; 12 int n,s,t,cnt; 13 int Head[N],Depth[N],cur[N],Map[N][N]; 14 int Next[M],V[M],W[M]; 15 16 void init(){ 17 cnt=-1; 18 memset(Head,-1,sizeof(Head)); 19 memset(Next,-1,sizeof(Next)); 20 } 21 22 void add_edge(int u,int v,int w){ 23 cnt++;Next[cnt]=Head[u];V[cnt]=v;W[cnt]=w;Head[u]=cnt; 24 cnt++;Next[cnt]=Head[v];V[cnt]=u;W[cnt]=0;Head[v]=cnt; 25 } 26 27 bool bfs(){ 28 queue <int> Q; 29 while(!Q.empty()) Q.pop(); 30 memset(Depth,0,sizeof(Depth)); 31 Depth[s]=1; 32 Q.push(s); 33 while(!Q.empty()){ 34 int u=Q.front();Q.pop(); 35 for(int i=Head[u];i!=-1;i=Next[i]){ 36 if((W[i]>0)&&(Depth[V[i]]==0)){ 37 Depth[V[i]]=Depth[u]+1; 38 Q.push(V[i]); 39 } 40 } 41 } 42 if(Depth[t]==0) return 0; 43 return 1; 44 } 45 46 int dfs(int u,int dist){ 47 if(u==t) return dist; 48 for(int& i=cur[u];i!=-1;i=Next[i]){ 49 if((Depth[V[i]]==Depth[u]+1)&&W[i]!=0){ 50 int di=dfs(V[i],min(dist,W[i])); 51 if(di>0){ 52 W[i]-=di; 53 W[i^1]+=di; 54 return di; 55 } 56 } 57 } 58 return 0; 59 } 60 61 int Dinic(){ 62 int ans=0; 63 while(bfs()){ 64 for(int i=0;i<=2*n;i++) cur[i]=Head[i]; 65 while(int d=dfs(s,INF)) ans+=d; 66 } 67 return ans; 68 } 69 70 struct TnT{ 71 double x,y; 72 int n,m; 73 }node[N]; 74 75 double dis(TnT a,TnT b){ 76 return (a.x-b.x)*(a.x-b.x)+(a.y-b.y)*(a.y-b.y); 77 } 78 79 int check(){ 80 for(int i=1;i<=n;i++) add_edge(s,i,node[i].n); 81 for(int i=1;i<=n;i++) add_edge(i,i+n,node[i].m); 82 for(int i=1;i<=n;i++) 83 for(int j=1;j<=n;j++) 84 if(Map[i][j]) add_edge(i+n,j,INF); 85 86 return Dinic(); 87 88 } 89 90 int main(){ 91 int T; 92 double d; 93 scanf("%d",&T); 94 while(T--){ 95 int sum=0; 96 scanf("%d %lf",&n,&d); 97 for(int i=1;i<=n;i++){ 98 scanf("%lf %lf %d %d",&node[i].x,&node[i].y,&node[i].n,&node[i].m); 99 sum+=node[i].n; 100 } 101 for(int i=1;i<=n;i++){ 102 for(int j=1;j<=n;j++){ 103 if(i==j) Map[i][j]=1; 104 else if(dis(node[i],node[j])<=d*d) Map[i][j]=1; 105 else Map[i][j]=0; 106 } 107 } 108 vector <int> ans; 109 for(int i=1;i<=n;i++){ 110 init();s=0;t=i; 111 if(check()==sum) ans.push_back(i); 112 } 113 int len=ans.size(); 114 if(len==0) printf("-1\n"); 115 else{ 116 for(int i=0;i<len;i++){ 117 if(i!=len-1) printf("%d ",ans[i]-1); 118 else if(i==len-1) printf("%d\n",ans[i]-1); 119 } 120 } 121 122 } 123 124 return 0; 125 }
POJ 3498 March of the Penguins(網絡流+枚舉)