【LeetCode】48. Rotate Image(C++)
阿新 • • 發佈:2018-12-02
地址:https://leetcode.com/problems/rotate-image/
題目:
You are given an n x n 2D matrix representing an image.
Rotate the image by 90 degrees (clockwise).
Note:
You have to rotate the image in-place, which means you have to modify the input 2D matrix directly. DO NOT allocate another 2D matrix and do the rotation.
Example 1
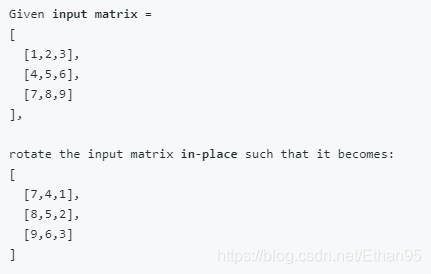
Example 2:
理解:
把圖片順時針旋轉。
可以先翻轉,再轉置。
實現:
class Solution { public: void rotate(vector<vector<int>>& matrix) { reverse(matrix.begin(),matrix.end()); for(int i=0;i<matrix.size();++i){ for(int j=i+1;j<matrix.size();++j) swap(matrix[i][j],matrix[j][i]); } } };
實現2:
考慮
a b
d c
把左上角的和其他三個位置分別swap也可以
b a
d c
c a
d b
d a
c b
需要確定的就是四個元素的下標。
相當於本次內迴圈完成最外層正方形的旋轉,內層由下一個外迴圈中的內迴圈完成。
class Solution { public: void rotate(vector<vector<int>>& matrix) { int m = 0, n = matrix.size() - 1; while (m < n) { for (int i = 0; i < (n - m); ++i) { swap(matrix[m][m + i], matrix[m + i][n]); swap(matrix[m][m + i], matrix[n][n - i]); swap(matrix[m][m + i], matrix[n - i][m]); } ++m; --n; } } };