JavaScript刷LeetCode -- 331. Verify Preorder Serialization of a Binary Tree
一、題目
One way to serialize a binary tree is to use pre-order traversal. When we encounter a non-null node, we record the node’s value. If it is a null node, we record using a sentinel value such as #.
_9_ / \ 3 2 / \ / \ 4 1 # 6 / \ / \ / \ # # # # # #
For example, the above binary tree can be serialized to the string “9,3,4,#,#,1,#,#,2,#,6,#,#”, where # represents a null node.
Given a string of comma separated values, verify whether it is a correct preorder traversal serialization of a binary tree. Find an algorithm without reconstructing the tree.
Each comma separated value in the string must be either an integer or a character ‘#’ representing null pointer.
You may assume that the input format is always valid, for example it could never contain two consecutive commas such as “1,3”.
二、題目大意
給定二叉樹的前序遍歷字串,驗證該字串是否有效,(空節點採用’#'表示)。
三、解題思路
這道題參考了社群大神的解法,簡單的理解就是:在遍歷的過程中,如果系統的入度大於出度,那麼“空閒分支”已經用完,後續節點無法掛載在二叉樹上,從而判斷字串是否有效。
四、程式碼實現
const isValidSerialization = preorder => {
const a = preorder.split(',')
let diff = 1
for (let item of a) {
diff -= 1
if (diff < 0) {
return false
}
if (item !== '#') {
diff += 2
}
}
return diff === 0
}
如果本文對您有幫助,歡迎關注微信公眾號,為您推送更多內容,ε=ε=ε=┏(゜ロ゜;)┛。
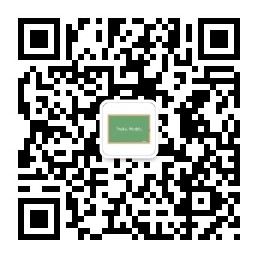