3.2 標準庫string型別
阿新 • • 發佈:2019-02-16
本章大意
講述了標準庫string型別的相關知識
細節摘錄
1. 字串字面值和string型別是不同的型別,這點要明確。別讓它們相加。
2. string物件定義了許多比較實用的操作。比如emptysize等。
3. 儲存string的size操作結果的變數必須為string::size_type,不要給int。
4. 在cctype標頭檔案中定義了一組操作可以對字串進行一些判斷。
5. 用string型別記得包含string標頭檔案。
課後習題
1. 預設建構函式是指讓構造一個物件且並不給出引數時系統自動執行的建構函式。
2. 經典的四種賦值方法如下:
4. 每次讀取一行的程式碼:
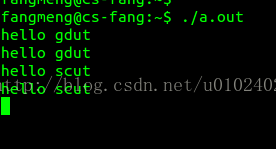
每次讀取一個單詞的程式碼:
5. cin省略掉開頭空白符,geilne則不省略。最後的換行則都會省略掉。
6. 程式碼如下:
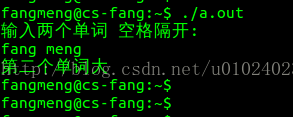
7. 直接用+運算即可。和第6題一樣,string成了一個真正意義上的“自定義資料”。
8. 合法。因為s中根本一個元素都沒有,而s[0]則試圖去讀取第一個元素。
9. 程式碼:
講述了標準庫string型別的相關知識
細節摘錄
1. 字串字面值和string型別是不同的型別,這點要明確。別讓它們相加。
2. string物件定義了許多比較實用的操作。比如emptysize等。
3. 儲存string的size操作結果的變數必須為string::size_type,不要給int。
4. 在cctype標頭檔案中定義了一組操作可以對字串進行一些判斷。
5. 用string型別記得包含string標頭檔案。
課後習題
1. 預設建構函式是指讓構造一個物件且並不給出引數時系統自動執行的建構函式。
2. 經典的四種賦值方法如下:
3. s和s2都是空字串string s1; // 預設建構函式,s1為空串。 string s2(s1); // 將s2初始化為s1的一個副本。 string s3("value"); // 將s3初始化為一個字串字面值副本。 string s4(n, 'c'); // 將s4初始化為字元'c'的n個副本。
4. 每次讀取一行的程式碼:
#include <iostream>
using namespace std;
int main()
{
string line;
/* 記住,getline並不忽略開頭的換行符。 */
while (getline(cin, line)) {
cout << line;
}
return 0;
}
執行結果每次讀取一個單詞的程式碼:
執行結果略#include <iostream> using namespace std; int main() { string s; while (cin >> s) { cout << s << endl; } return 0; }
5. cin省略掉開頭空白符,geilne則不省略。最後的換行則都會省略掉。
6. 程式碼如下:
執行結果#include <iostream> #include <string> using namespace std; int main() { string s1, s2; cout << "輸入兩個單詞 空格隔開: " << endl; cin >> s1 >> s2; if (s1 > s2) { cout << "第一個單詞大" << endl; } else if (s1 < s2) { cout << "第二個單詞大" << endl; } else { cout << "兩個單詞相等" << endl; } return 0; }
7. 直接用+運算即可。和第6題一樣,string成了一個真正意義上的“自定義資料”。
8. 合法。因為s中根本一個元素都沒有,而s[0]則試圖去讀取第一個元素。
9. 程式碼:
#include <iostream>
#include <string>
using namespace std;
int main()
{
string s1, s2;
cout << "請輸入待處理字串:" << endl;
cin >> s1;
for (string::size_type i=0; i<s1.length(); i++) {
if (!ispunct(s1[i])) {
s2 += s1[i];
}
}
cout << "處理後:" << s2 << endl;
return 0;
}